It is the transformation animations that are performed by some method or function.
Class | Parameters | Explanation |
ApplyMethod | mobject, *args, **kwargs |
Animation effect where a method of mobject is performed. The *args is the parameter for method, and *8kwargs is CONFIG parameter dictionary for the ApplyMethod. |
ApplyPointwiseFunction | function, mobject, **kwargs |
Animate the transformation by applying a function to the object. Where function is the operation for each point of mobject. |
ApplyFunction | function, mobject, **kwargs |
Animate the transformation by applying a function to the object. function must return an Mobject object |
Homotopy | homotopy, mobject, **kwargs |
Animation applying homotopy function to mobject. The homotopy function is (x,y,z,t) -> (x', y', z) |
PhaseFlow | function, mobject, **kwargs |
Animation that applies a function that is a function of time t to mobject |
ApplyMethod class
object > Animation > Transform > ApplyMethod
manimlib.animation.transform.ApplyMethod(self, method, *args, **kwargs)
Animation effect where a method of mobject is performed.
The *args is the parameter for method, and **kwargs is CONFIG parameter dictionary for the ApplyMethod.
self.play(ApplyMethod(text.scale, 1.5)) # animation that enlarge the text
Parameters: method
Method of the mobject.
Parameters: *args
Parameters for the method
Parameters: **kwargs
CONFIG values of Transform/Animation
Frequently used variables are,
- run_time=1.0: Animation running time
- rate_func=smooth: Velocity change function when the animation is running
Mobject has several methods to change the position/property of the object, a method to change the position such as to_edge and a method to change the color such as set_color.
The class that animates the execution of this method is ApplyMethod.
The example below animates sending the left arrow to the right with the to_edge(RIGHT) method.
arrow = Arrow().to_edge(LEFT)
self.add(arrow)
self.play(ApplyMethod(arrow.to_edge, RIGHT))
self.wait()
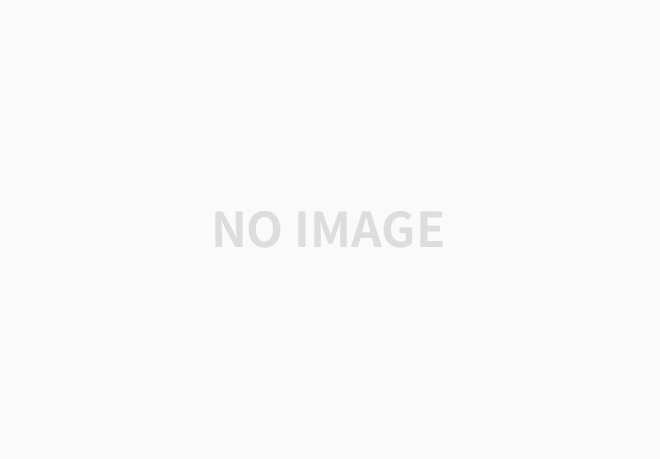
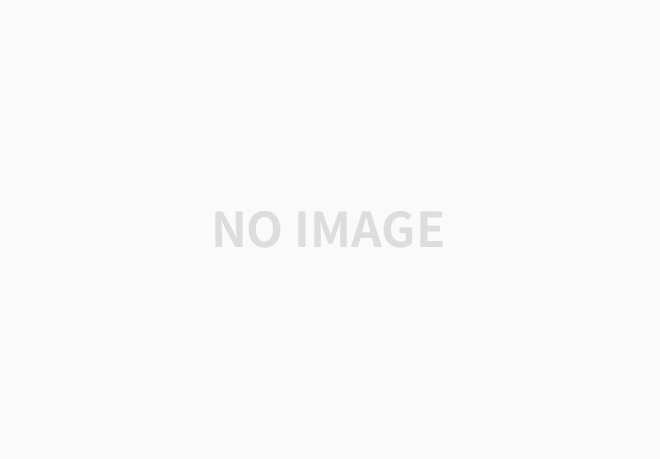
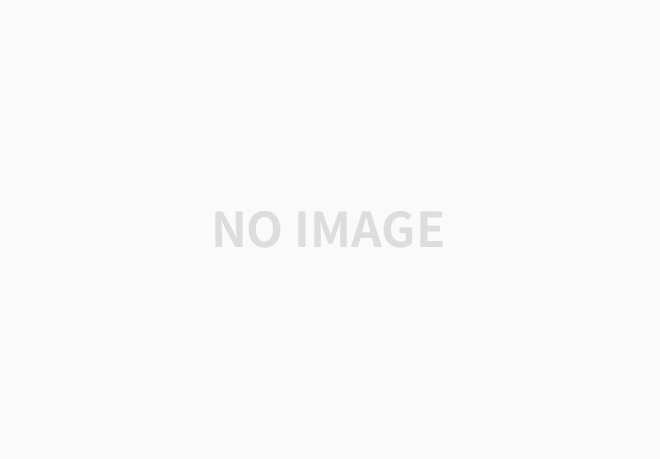
ApplyPointwiseFunction class
object > Animation > Transform > ApplyMethod > ApplyPointwiseFunction
manimlib.animation.transform.ApplyPointwiseFunction(self, function, mobject, **kwargs)
Animate the transformation by applying a function to the object. Where function is the operation for each point of mobject.
CONFIG = {
"run_time": DEFAULT_POINTWISE_FUNCTION_RUN_TIME
}
Parameters: function
Function for the each point of the mobject
Parameters: mobject
Mobject instance to be target
Parameters: **kwargs
CONFIG value of ApplyPointwiseFunction and Transform/Animation
Frequently used variables are,
- run_time=1.0: Animation running time
- rate_func=smooth: Velocity change function when the animation is running
This animation is used to change each point of the object through a function and to animate this function execution.
For example, you can create an animation where a small circle is in the center of the screen and each point in the circle gets larger and wider.
def apply_pointwise_function(self):
circle = Circle(radius=0.5)
circle.save_state()
self.add(circle)
self.wait()
self.play(
ApplyPointwiseFunction(
lambda p: 10 * p / get_norm(p),
circle
)
)
self.play(Restore(circle))
circle.set_fill(color=RED, opacity=0.5)
self.play(
ApplyPointwiseFunction(
lambda p: 10 * p / get_norm(p),
circle
)
)
self.wait(2)
ApplyFunction class
object > Animation > Transform > ApplyFunction
manimlib.animation.transform.ApplyFunction(self, function, mobject, **kwargs)
Animate the transformation by applying a function to the object. function must return an Mobject object
The difference is that ApplyMethod animates the execution of the methods that Mobject has, whereas ApplyFunction animates a newly created function.
In other words, ApplyMethod applies to the existing method, and ApplyFunction applies the newly created function rather than the existing method.
Parameters: function
Function of Mobject
Parameters: mobject
Object to be target
Parameters: **kwargs
CONFIG values of Transform/Animation
Frequently used variables are,
- run_time=1.0: Animation running time
- rate_func=smooth: Velocity change function when the animation is running
Instead of the methods inherent in the Mobject object, we can apply our new function to the object.
The new function must return the changed object after moving the object, changing the color, etc.
The example below is an animation that fades out a circle and slowly descends.
def apply_function(self):
circle = Circle(fill_opacity=1, color=RED).shift(DOWN)
circle.save_state()
self.add(circle)
self.wait()
self.play(
ApplyFunction(
lambda m : m.fade(1).shift(5*DOWN),
circle,
run_time=6,
)
)
self.wait(2)
Homotopy class
object > Animation > Homotopy
manimlib.animation.movement.Homotopy(self, homotopy, mobject, **kwargs)
Animation applying homotopy function to mobject.
The homotopy function is (x,y,z,t) -> (x', y', z)
CONFIG = {
"run_time": 3,
"apply_function_kwargs": {},
}
Parameters: homotopy
Homotopy function: continuous transforming function
This function change (x, y, z, t) to (x', y', z')
Parameters: mobject
Mobject instance to be target
Parameters: **kwargs
CONFIG values of Homotopy and Animation
Frequently used variables are,
- run_time=1.0: Animation running time
- rate_func=smooth: Velocity change function when the animation is running
This animation is mainly used for animations that make objects swing up and down or left and right. To make it sway left and right, you can change the x-axis value according to t as shown in the code below.
def homotopy_x(x, y, z, t):
alpha = (0.7*x + FRAME_Y_RADIUS)/(FRAME_HEIGHT)
beta = squish_rate_func(smooth, alpha-0.15, alpha+0.15)(t)
return (x - 0.3*np.sin(np.pi*beta), y, z)
To make it sway left and rigth, you can change the x-vaxis value according to t as shown in the code below.
def homotopy_x(x, y, z, t):
alpha = (0.7*x + FRAME_Y_RADIUS)/(FRAME_HEIGHT)
beta = squish_rate_func(smooth, alpha-0.15, alpha+0.15)(t)
return (x - 0.3*np.sin(np.pi*beta), y, z)
To make it sway up and down, you can apply a function whose y value change with t.
def homotopy_y(x, y, z, t):
alpha = (0.7*x + FRAME_X_RADIUS)/(FRAME_WIDTH)
beta = squish_rate_func(smooth, alpha-0.15, alpha+0.15)(t)
return (x, y - 0.3*np.sin(np.pi*beta), z)
In the example below, the above functions are applied to create a sloopy effect on the objects.
def homotopy(self):
def homotopy_x(x, y, z, t):
alpha = (0.7*x + FRAME_Y_RADIUS)/(FRAME_HEIGHT)
beta = squish_rate_func(smooth, alpha-0.15, alpha+0.15)(t)
return (x - 0.3*np.sin(np.pi*beta), y, z)
def homotopy_y(x, y, z, t):
alpha = (0.7*x + FRAME_X_RADIUS)/(FRAME_WIDTH)
beta = squish_rate_func(smooth, alpha-0.15, alpha+0.15)(t)
return (x, y - 0.3*np.sin(np.pi*beta), z)
text = TextMobject("Homotopy Animation")
circle = Circle()
square = Square()
objs = VGroup(text, circle, square).arrange(RIGHT, buff=1)
self.add(objs)
self.wait()
for i in range(0, 2):
self.play(Homotopy(homotopy_x, objs))
for i in range(0, 2):
self.play(Homotopy(homotopy_y, objs))
self.wait()
PhaseFlow class
object > Animation > PhaseFlow
manimlib.animation.movement.PhaseFlow(self, function, mobject, **kwargs)
Animation that applies a function that is a function of time t to mobject
CONFIG = {
"virtual_time": 1,
"rate_func": linear,
"suspend_mobject_updating": False,
}
Parameters: function
Function varying according to the time
Parameters: mobject
Mobject instance to be target
Parameters: **kwargs
CONFIG values of PhaseFlow and Animation
Frequently used variables are,
- run_time=1.0: Animation running time
- rate_func=smooth: Velocity change function when the animation is running
The example below is an naimation that spreads the NumberPlane and the objects contained in it wide from side side over time.
def phase_flow(self):
name_text = Text("PhaseFlow", stroke_width=0, size=0.4, color=YELLOW)
name_text.to_corner(UL)
plane = NumberPlane(color=BLUE_E)
square = Circle(color=YELLOW)
plane.add(square)
self.add(plane, name_text)
self.wait()
def func(t):
return t * 0.1 * RIGHT
self.play(PhaseFlow(
func, plane,
run_time=5,
virtual_time=8,
))
Next: [06-1-H] Animation : Grouping
[06-1-H] Animation : Grouping
Animations can be grouped and handled. When grouped, whether to run each animation together or one by one is determined by lag_ratio. lag_ratio = 0 : Run together (simultaneously) lag_ratio = 1: The..
infograph.tistory.com
Go To: [99] Table of Contents
'Programming > Manim Lectures' 카테고리의 다른 글
[06-1-I] Animation : Special Effect (0) | 2020.06.09 |
---|---|
[06-1-H] Animation : Grouping (0) | 2020.06.09 |
[06-1-F] Animation : Transformation series (2/3) (0) | 2020.06.09 |
[06-1-E] Animation : Transformation series (1/3) (0) | 2020.06.09 |
[06-1-D] Animation : Move/Rotating series (0) | 2020.06.09 |